RPA¶
The Robotic Process Automation python script is generated based on the low-level most frequent routine, after the user had the opportunity to edit some fields.
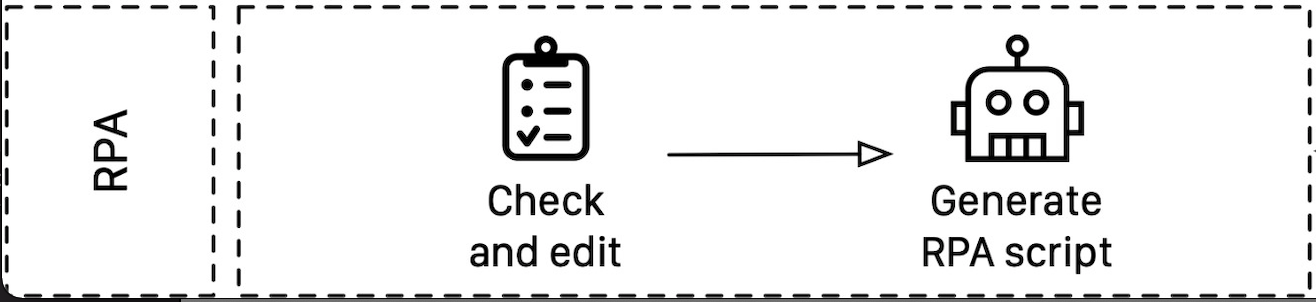
Check and edit event log¶
Explained in Choices dialog.
Python RPA script¶
- class modules.RPA.generateRPAScript.RPAScript(csv_file_path: str, status_queue: Queue, delay_between_actions=0.2)[source]¶
This class generate python RPA SW robot for a given csv
- __init__(csv_file_path: str, status_queue: Queue, delay_between_actions=0.2)[source]¶
Initialize class and import csv event log into dataframe
- Parameters:
csv_file_path – path of event log to analyze
status_queue – queue to sent messages to GUI
delay_between_actions – delay between robot actions in milliseconds
- static _createBrowserHeader()[source]¶
Create import statement for the browser (import selenium for automation).
It is separated because those imports are added only if there are browser events.
- Returns:
browser import statement
- _createHeader()[source]¶
Add import statements to generated python file with required packages like automagica
- Returns:
import statement
- _createOpenExcel(script, path)[source]¶
Write instructions to open excel.
- Parameters:
script – file object to write to
path – path of excel file to open
- _createRPAFile(RPA_type)[source]¶
Generate path where to save python SW robot
- Parameters:
RPA_type – name of file
- Returns:
path of SW robot, like like /Users/marco/Desktop/ComputerLogger/RPA/2020-02-25_23-21-57/2020-02-25_23-21-57_RPA.py
- _generateUnifiedRPA(df: DataFrame, filename='_UnifiedRPA.py')[source]¶
Core method of the class, generates python SW robot from trace.
Each line of the dataframe is analyzed and the corresponding python instruction to automate it is generated.
- Parameters:
df – low-level dataframe of trace to automate
filename – name of resulting sw robot
UiPath RPA project¶
Note
The primary methods of this class to work with are:
generateUiPathRPA() - method called to start UiPath SW Robot generation process
__generateRPA() - Main method to generate UiPath SW robot
__generateActivities() - convert an event into a UiPath XML node
- class modules.RPA.uipath.UIPathXAML(csv_file_path: str, status_queue: Queue, df: DataFrame)[source]¶
Automatically generate RPA script compatible with UiPath. Called by GUI when main process is terminated and csv is available.
- __attachBrowser(activities: list)¶
Attach browser element. This sequence contains browser events and it attaches to the OpenBrowser sequence created at the beginning. It is used every time there are browser events.
- Parameters:
activities – list of activities to append
- Returns:
Attach browser element
- __click(xpath: str, xpath_full: str, clickType='CLICK_SINGLE', mouseButton='BTN_LEFT', displayName: str = 'Click Item')¶
Element to click on web objects
- Parameters:
xpath – xpath of web element
xpath_full – full xpath of web element
clickType – type of click (single, double click)
mouseButton – button of mouse to click (left, right, middle)
displayName – name of click element in uipath
- Returns:
Click element
- __closeApplication(app: str, title: str, displayName: str = 'Start Process')¶
Element to close an open application
- Parameters:
app – name of the application to close
title – title of the application window
displayName – name of the element in UiPath
- Returns:
CloseApplication element
- __closeTab()¶
Element to close tab
- Returns:
closeTab element
- __closeWorkbook(displayName: str = 'Close Workbook')¶
Close opened workbook
- Parameters:
displayName – name of element in UiPath
- Returns:
ExcelCloseWorkbook element
- __comment(text: str)¶
Create comment element :param text: text inside the comment :return: append comment to main sequence
- __createDirectory(path: str, displayName: str = 'Create file')¶
Element to create a directory
- Parameters:
path – path of directory to be created
displayName – name of element in UiPath
- Returns:
CreateDirectory element
- __createFile(path: str, displayName: str = 'Create file')¶
Element to create a file
- Parameters:
path – path of file to be created
displayName – name of element in UiPath
- Returns:
CreateFile element
- __createMainSequence()¶
Create main sequence that will contain all other sequences and events and append it to root.
- __createOpenBrowser(df: DataFrame)¶
Method to insert openBrowser element at the beginning of the XAML file if browser events are present in the event log.
- Parameters:
df – input dataframe
- __createOpenExcel(df: DataFrame)¶
Method to insert openExcel element at the beginning of the XAML file if excel events are present in the event log.
- Parameters:
df – input dataframe
- __createRoot()¶
Generate XML root with namespace declarations. The base XML file for UiPath starts here.
reference https://stackoverflow.com/a/31074030
- __createSequence(activities: list, displayName: str = 'Do', key=None)¶
Create UiPath sequence element, which is a container for other elements.
- Parameters:
activities – list of activities inside the sequence
displayName – name of the sequence
key –
- Returns:
sequence
- __createTextExpression()¶
Append required text expressions to XML root
- __delete(path: str, displayName: str = 'Delete file')¶
Element to delete a file/folder
- Parameters:
path – path where te file/folder is
displayName – name of element in UiPath
- Returns:
Delete element
- __df_without_duplicates()¶
Filter unsupported events from dataframe, namely events that
- Returns:
dataframe without unsupported events
Deprecated since version 1.2.0: Not in use anymore since decision point analysis now occurs before RPA script generation.
- __excelSpreadsheet(workbookPath: str = '', password: str = '{x:Null}', attach=True, displayName: str = 'Excel Application Scope', activities: Optional[list] = None)¶
Element to handle excel spreadsheets.
- Parameters:
workbookPath – path of workbook to open
password – workbook password (if present)
attach – if True, attaches to a workbook already opened by UiPath, without opening it again
displayName – name of element in UiPAth
activities – list of activities to perform on opened excel workbook
- Returns:
ExcelApplicationScope element
- __generateActivities(df: DataFrame, row: Series)¶
Helper method called for each row of the dataframe to convert an event into a UiPath XML node.
- Parameters:
df – input dataframe
row – current row of dataframe
- Returns:
XML node
- __generateRPA(df: DataFrame)¶
Main method to generate UiPath SW robot.
The main steps are:
Add comment with SmartRPA link to UiPath file
Create open browser and open excel activities if the corresponding events are present in the dataframe
create activities dictionary to store XML nodes divided by category.
For each row in the dataframe:
generate XML node from event
append node to activities dictionary based on its categoru
when there is a change in category of in the last loop iteration, write nodes to main XAML sequence
- Parameters:
df – input dataframe of trace to automate
- __generateTraceKeywords(options: list)¶
Used to generate keywords dataframe to explain different decision to user.
Not in use anymore since decision point analysis now occurs before RPA script generation.
- Parameters:
options – list of traces
- Returns:
keywords dataframe
Deprecated since version 1.2.0: Not in use anymore since decision point analysis now occurs before RPA script generation.
- __getIdFromXpath(xpath: str)¶
Get ID of element from xpath
- Parameters:
xpath – xpath of web element
- Returns:
id of element
- __getIdxFromXpath(a: str, b: str, list_index: int)¶
Compare current xpath to previous one to find differences in index.
Since XPATH selection is not supported by UiPath, CSS selection is used. With this method, web elements are identified using their relative index on the page.
This method calculates the index of an element relative to another.
For example, in a google form, 3 consecutive text field have index 0 to 3.
- Parameters:
a – xpath of first element
b – xpath of second element
list_index – list of xpath column in dataframe
- Returns:
index of current element
- __howManyDecisionVariables()¶
return number of groups that will be converted into switch statements in UiPath used to determine how many variables should be added to main sequence
- Returns:
number of decision points
Deprecated since version 1.2.0: Not in use anymore since decision point analysis now occurs before RPA script generation.
- __init__(csv_file_path: str, status_queue: Queue, df: DataFrame)[source]¶
- Parameters:
csv_file_path – path of event log csv
status_queue – queue to print messages on GUI
df – dataframe of a trace of execution to automate
- __inputDialog(options: list, label: Optional[str] = None, title: str = 'Decision point', displayName: Optional[str] = None)¶
Create input dialog element
- Parameters:
options – list of options to display in dialog
label – label of input dialog
title – title of input dialog
displayName – name of input dialog
- Returns:
input dialog
Deprecated since version 1.2.0: Not in use anymore since decision point analysis now occurs before RPA script generation.
- __insertSlide(position)¶
Add slides to a presentation.
- Parameters:
position – where to add slides
- Returns:
InsertSlideX element
- __moveFile(from_path: str, to_path: str, displayName: str = 'Move file')¶
Element to move a file/folder
- Parameters:
from_path – path where te file/folder is
to_path – destination path where to move the file/folder
displayName – name of element in UiPath
- Returns:
MoveFile element
Element to navigate on a page
- Parameters:
url – url to open
displayName – name of element in UiPath
- Returns:
navigateTo element
- __openApplication(arguments: str = '{x:Null}', fileName: str = '{x:Null}', selector: str = '{x:Null}', displayName: str = 'Open Application', activities: Optional[list] = None)¶
element to open specified application
- Parameters:
arguments – path of program to open
fileName – name of program to open
selector – windows selector
displayName – name of element in UiPath
activities – list of activities
- Returns:
OpenApplication element
- __openBrowser(url: str = '', activities: Optional[list] = None)¶
Open browser. This sequence contains browser events and it’s used only once at the beginning of the project.
- Parameters:
url – open at specific url
activities – list of activities to append
- Returns:
append open browser sequence to main sequence
- __openFileFolder(path: str, itemName: str)¶
open file or folder in windows explorer
- Parameters:
path – path of file or folder to open
itemName – name of file or folder to open
- Returns:
OpenApplication element
- __powerpointScope(num_slides: int, path: str = '', displayName: str = 'PowerPoint Presentation')¶
Element to handle powerpoint events.
- Parameters:
num_slides – number of slides to add to the presentation
path – path of powerpoint presentation. If empty create a new file.
displayName – name of element in UiPath
- Returns:
PowerPointApplicationCard element
- __saveWorkbook(displayName: str = 'Save Workbook')¶
Save opened workbook
- Parameters:
displayName – name of element in UiPath
- Returns:
ExcelSaveWorkbook element
- __sendHotkey(key: str = '{x:Null}', modifiers: str = 'None', displayName: str = 'Send Hotkey')¶
Element to type specified sequence of keys
- Parameters:
key – letter to press
modifiers – modifiers to press (like CTRL, ALT, etc)
displayName – name of element in UiPath
- Returns:
SendHotkey element
- __setToClipboard(text: str, displayName: str = 'Set to clipboard')¶
Set specified text to clipboard
- Parameters:
text – text to be set to clipboard
displayName – name of element in UiPath
- Returns:
SetToClipboard element
- __startProcess(path: str, displayName: str = 'Start Process')¶
Element to start specified process
- Parameters:
path – path of process to start
displayName – name of element in UiPath
- Returns:
StartProcess element
- __switch(caseActivities: dict, defaulActivity=None, condition=None, displayName: str = 'Switch')¶
Create switch element. Before each switch, an input dialog is needed to ask the user which case to choose
- Parameters:
caseActivities – dictionary of activities divided by trace
defaulActivity – default case to execute
condition – switch condition
displayName – name of switch element
- Returns:
switch element
Deprecated since version 1.2.0: Not in use anymore since decision point analysis now occurs before RPA script generation.
- __target(xpath: str = '', xpath_full: str = '', idx: str = '', app: str = '', title: str = '', timeout: str = '1000')¶
Target element to click on.
- Parameters:
xpath – xpath of web element
xpath_full – full xpath of web element
idx – index of element in page
app – name of the browser used to navigate (e.g. google chrome)
title – title of the window of the browser
timeout – timeout after which action is dismissed if element is not found
- Returns:
target element
- __typeInto(text: str, xpath: str = '', xpath_full: str = '', idx: str = '', app: str = '', title: str = '', displayName: str = "Type into 'INPUT'", emptyField: bool = True, timeout: str = '1000', newLine: bool = False)¶
TypeInto element to type into web fields.
Uses __target() method to create target element.
- Parameters:
text – text to be typed
xpath – xpath of web element
xpath_full – full xpath of web element
idx – index of web element
app – name of the program used to navigate (e.g. google chrome)
title – title of the window of the browser
displayName – name of TypeInto element
emptyField – if True, field is empied before typing
timeout – timeout after which action is dismissed if element is not found
newLine – add new line after typing
- Returns:
TypeInto element
- __writeCell(cell: str, sheetName: str, text: str)¶
Write excel cell
- Parameters:
cell – position cell to write
sheetName – name of sheet
text – text to write
- Returns:
ExcelWriteCell element
- __xpathToCssSelector(xpath: str)¶
Convert XPATH to CSS selector.
The action logger records the XPATH for each web element so it can easily be identified by the SW robot. UiPath does not support selection of web elements using XPATH (which is more accurate), so conversion to CSS selector is needed.
- Parameters:
xpath –
- Returns:
- createBaseFile()[source]¶
Create base XAML file for UiPath.
create root element
add text expressions
add main sequence